#3.10 Flexbox Part One (12:08) : justify-content
더보기

개괄
1. 자식에게 명시하지 않고 부모에게 명시한다
div의 부모를 display:flex로 만든다
2. main axis=> 주축은 (수평)이 기본, justify-content는 main axis를 따라 움직임
3. cross axis=> 교차축은 (수직)이 기본, align-items는 cross axis를 따라 움직임
4. body가 height 값을 갖고있지 않으면 이미 맨 위아래를 차지하고 중심에 있으니까 align-items를 설정하더라도 바뀌지 않음.
이 때 height를 px로 해도 작동하지만, vh를 사용하면 화면 크기에 따라 바뀐다. 아이폰 맥북 아이패드에 따라 다 달라지니까 유용하다.

justify-content
justify-content
- space-evenly : 균형있게 배치
- space-around : 균형있게 배치하되 처음과 끝요소의 각 바깥쪽 여백을 1/2로 함
- space-between : 균형있게 배치하되 처음과 끝 요소를 각 border에 위치
- flex-start : 앞쪽으포 배치
- flex-end : 끝으로 배치
- center : 가운데 배치
실전 코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
margin: 20px;
display: flex;
height: 100vh;
/* 100%의미 */
justify-content: space-around;
align-items: center;
}
div {
width: 300px;
margin: 3px;
height: 300px;
background: teal;
}
#second {
background: wheat;
}
</style>
</head>
<body>
<div></div>
<div id="second"></div>
<div></div>
</body>
</html>
#3.11 Flexbox Part Two (09:02) : flex-wrap / flex-direction
더보기

개괄
direction
- justify-content나 align-items의 default를 변경하기 위해선, 'flex-direction'을 수정하면 된다.
- flex-direction에는 두 가지 속성, column과 row가 있다.
- display를 flex로 했을 때 default는 row이다. 따라서 flex-direction: column;을 주면 주축과 교차축이 반전된다.
- 원하는만큼 flex 부모-자식 엘리먼트를 만들어낼 수 있다.
- flex-direction: column-reverse; 밑에서 시작해서 위로 올라가게 한다.(마찬가지로 row-reverse도 있다.)
wrap
- flex-wrap: nowrap;을 통해 wrapping이 일어나지 않게 할 수 있다.
- flexbox는 width값을 초기 사이즈로만 여기고, 모든 엘리먼트를 같은 줄에 있게 하기 위해 width를 바꾸기도 한다.
- flex-wrap : wrap; 한줄에 콘텐츠가 들어갈 수 있을 만큼만 보여주고 나머지는 다음줄로 넘김
- flex-wrap: wrap-reverse; 또한 있는데, 브라우저를 줄일 때, 엘리먼트가 겹쳐지는 위치가 역전된다.
실습사이트
https://flexboxfroggy.com/#ko%EF%BB%BF
Flexbox Froggy
A game for learning CSS flexbox
flexboxfroggy.com
실습코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
margin: 20px;
display: flex;
height: 100vh;
/* 100%의미 */
flex-direction: column;
justify-content: space-around;
align-items: center;
flex-wrap: nowrap;
flex-direction: row-reverse;
}
div {
width: 300px;
margin: 3px;
height: 300px;
background: teal;
display: flex;
justify-content: center;
align-items: center;
}
#second {
background: wheat;
}
</style>
</head>
<body>
<div>1</div>
<div id="second">2</div>
<div>3</div>
</body>
</html>

#3.12 Fixed (08:19)
더보기

개괄
position: fixed
position: fixed하면 그 엘리먼트가 다른 layer에 위치하면서 layer의 맨 앞층으로 오고.
position: fixed 하기 전 위치에 고정됨
position: fixed만 사용했을때는 같은 레이어인 것처럼 보이나,
top, left, right, bottom의 프로퍼티 중 하나라도 이용해 해당 element의
위치를 임의로 변경시킨다면 원래 위치가 무시(block의 속성으로 순서대로 배치되는 위치)되는 것을 확인할 수 있음.
실습코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
height: 1000vh;
margin: 20px;
}
div {
width: 300px;
height: 300px;
background: wheat;
color: teal;
/* position: fixed; */
}
#wheat {
position: fixed;
}
#green {
background: teal;
width: 350px;
position: fixed;
/* top left bottom right : 초기위치(block)이 겹치지 않으려고 순서대로 위치)를 무시하고 position의 위치를 조절할 수 있음 */
top: 5px;
/* position의 위치를 확인하기 위해 투명도 설정 */
opacity: 0.2;
}
</style>
</head>
<body>
<div id="wheat"></div>
<div id="green"></div>
</body>
</html>

#3.13 Relative Absolute (07:45)
더보기


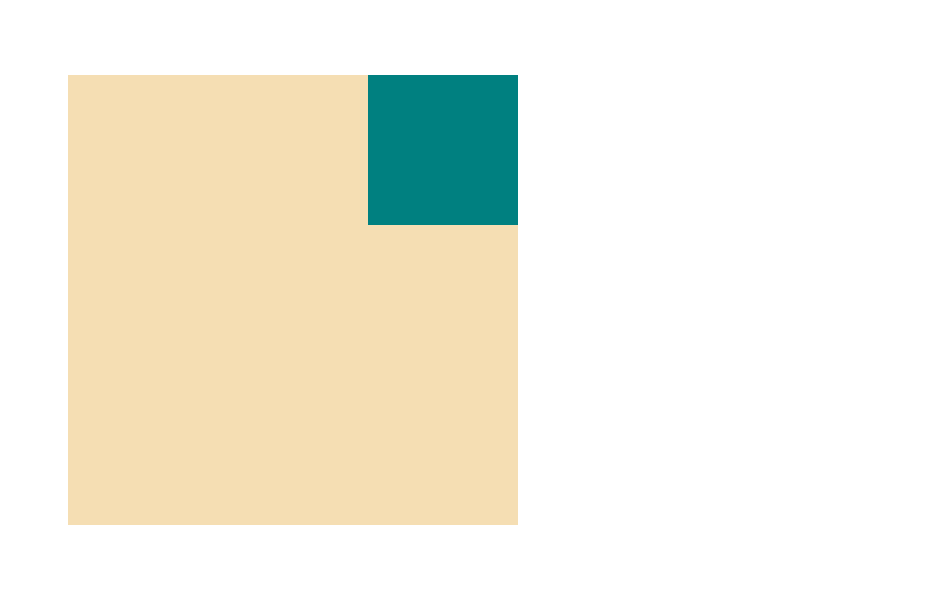
개괄
1. positon: static (default)
2. position: fixed
- element가 처음 생성된 자리에 고정.
3. position: relative;
- element가 '처음 생성된 위치'를 기준점으로, top bottom left right으로 위치를 조금씩 수정할 수 있다.
4. position: absolute;
가장 가까운 relative 부모를 기준으로 이동
position:relative; 를 해주면 부모가 된다.
없으면 body.
relative 실습코드 : 처음 생성된 위치를 기준으로
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
height: 1000vh;
margin: 50px;
}
div {
width: 300px;
height: 300px;
background: wheat;
color: teal;
position: relative;
}
.green {
background: teal;
height: 100px;
width: 100px;
top: -10px;
left: -10px;
}
</style>
</head>
<body>
<div>
<div class="green"></div>
</div>
</body>
</html>

position 실습코드 : 부모기준으로(가까운 relative가 부모, but relative가 없으면 body가 부모)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
height: 1000vh;
margin: 50px;
}
div {
width: 300px;
height: 300px;
background: wheat;
color: teal;
}
.green {
background: teal;
height: 100px;
width: 100px;
position: absolute;
right: 0px;
}
</style>
</head>
<body>
<div>
<div class="green"></div>
</div>
</body>
</html>

position 실습코드 : 부모기준으로(가까운 relative가 있으면 relative가 부모)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
height: 1000vh;
margin: 50px;
}
div {
width: 300px;
height: 300px;
background: wheat;
color: teal;
position: relative;
}
.green {
background: teal;
height: 100px;
width: 100px;
position: absolute;
right: 0px;
}
</style>
</head>
<body>
<div>
<div class="green"></div>
</div>
</body>
</html>
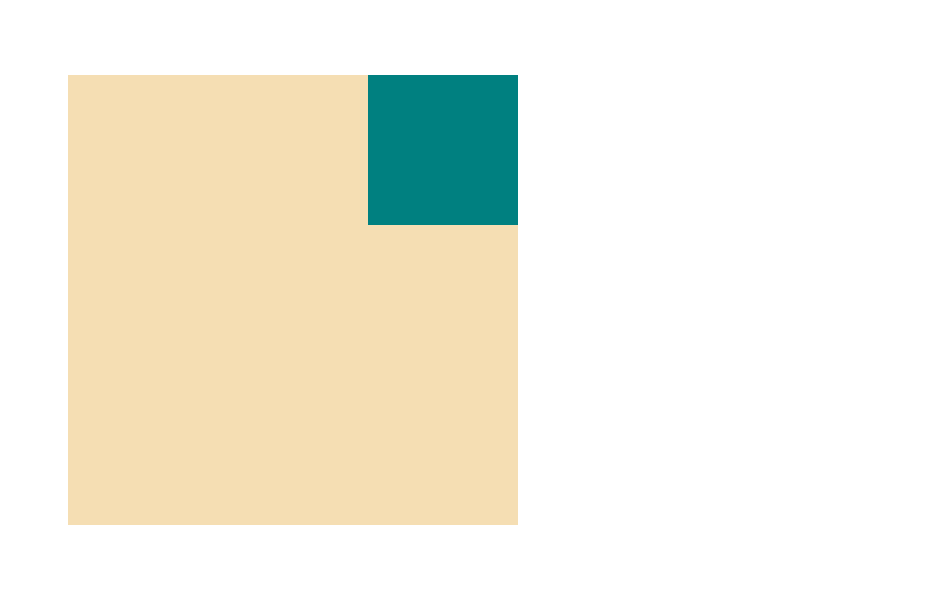